Box
Superclasses: Widget
, InitiallyUnowned
, Object
Subclasses: ShortcutsGroup
, ShortcutsSection
Implemented Interfaces: Accessible
, Buildable
, ConstraintTarget
, Orientable
The GtkBox
widget arranges child widgets into a single row or column.
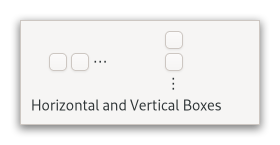
Whether it is a row or column depends on the value of its
orientation
property. Within the other
dimension, all children are allocated the same size. Of course, the
halign
and valign
properties
can be used on the children to influence their allocation.
Use repeated calls to append
to pack widgets into a
GtkBox
from start to end. Use remove
to remove widgets
from the GtkBox
. insert_child_after
can be used to add
a child at a particular position.
Use set_homogeneous
to specify whether or not all children
of the GtkBox
are forced to get the same amount of space.
Use set_spacing
to determine how much space will be minimally
placed between all children in the GtkBox
. Note that spacing is added
between the children.
Use reorder_child_after
to move a child to a different
place in the box.
CSS nodes
GtkBox
uses a single CSS node with name box.
Accessibility
Until GTK 4.10, GtkBox
used the GTK_ACCESSIBLE_ROLE_GROUP
role.
Starting from GTK 4.12, GtkBox
uses the GTK_ACCESSIBLE_ROLE_GENERIC
role.
Constructors
- class Box
- classmethod new(orientation: Orientation, spacing: int) Widget
Creates a new
GtkBox
.- Parameters:
orientation – the box’s orientation
spacing – the number of pixels to place by default between children
Methods
- class Box
- append(child: Widget) None
Adds
child
as the last child tobox
.- Parameters:
child – the
GtkWidget
to append
- get_baseline_child() int
Gets the value set by
set_baseline_child()
.Added in version 4.12.
- get_baseline_position() BaselinePosition
Gets the value set by
set_baseline_position()
.
- get_spacing() int
Gets the value set by
set_spacing()
.
- insert_child_after(child: Widget, sibling: Widget | None = None) None
Inserts
child
in the position aftersibling
in the list ofbox
children.If
sibling
isNone
, insertchild
at the first position.- Parameters:
child – the
GtkWidget
to insertsibling – the sibling after which to insert
child
- prepend(child: Widget) None
Adds
child
as the first child tobox
.- Parameters:
child – the
GtkWidget
to prepend
- remove(child: Widget) None
Removes a child widget from
box
.The child must have been added before with
append
,prepend
, orinsert_child_after
.- Parameters:
child – the child to remove
- reorder_child_after(child: Widget, sibling: Widget | None = None) None
Moves
child
to the position aftersibling
in the list ofbox
children.If
sibling
isNone
, movechild
to the first position.- Parameters:
child – the
GtkWidget
to move, must be a child ofbox
sibling – the sibling to move
child
after
- set_baseline_child(child: int) None
Sets the baseline child of a box.
This affects only vertical boxes.
Added in version 4.12.
- Parameters:
child – a child, or -1
- set_baseline_position(position: BaselinePosition) None
Sets the baseline position of a box.
This affects only horizontal boxes with at least one baseline aligned child. If there is more vertical space available than requested, and the baseline is not allocated by the parent then
position
is used to allocate the baseline with respect to the extra space available.- Parameters:
position – a
GtkBaselinePosition
Properties
- class Box
- props.baseline_child: int
The child that determines the baseline, in vertical orientation.
Added in version 4.12.
- props.baseline_position: BaselinePosition
The position of the baseline aligned widgets if extra space is available.
Fields
- class Box
- parent_instance