Switch
Superclasses: Widget
, InitiallyUnowned
, Object
Implemented Interfaces: Accessible
, Actionable
, Buildable
, ConstraintTarget
GtkSwitch
is a “light switch” that has two states: on or off.
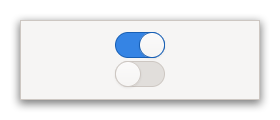
The user can control which state should be active by clicking the empty area, or by dragging the handle.
GtkSwitch
can also handle situations where the underlying state
changes with a delay. In this case, the slider position indicates
the user’s recent change (as indicated by the active
property), and the color indicates whether the underlying state (represented
by the state
property) has been updated yet.

See state_set
for details.
CSS nodes
switch
├── image
├── image
╰── slider
GtkSwitch
has four css nodes, the main node with the name switch and
subnodes for the slider and the on and off images. Neither of them is
using any style classes.
Accessibility
GtkSwitch
uses the SWITCH
role.
Constructors
Methods
Properties
Signals
- class Switch.signals
- activate() None
Emitted to animate the switch.
Applications should never connect to this signal, but use the
active
property.
- state_set(state: bool) bool
Emitted to change the underlying state.
The ::state-set signal is emitted when the user changes the switch position. The default handler keeps the state in sync with the
active
property.To implement delayed state change, applications can connect to this signal, initiate the change of the underlying state, and call
set_state
when the underlying state change is complete. The signal handler should returnTrue
to prevent the default handler from running.Visually, the underlying state is represented by the trough color of the switch, while the
active
property is represented by the position of the switch.- Parameters:
state – the new state of the switch